Notice
Recent Posts
Recent Comments
Link
일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | 2 | 3 | 4 | 5 | ||
6 | 7 | 8 | 9 | 10 | 11 | 12 |
13 | 14 | 15 | 16 | 17 | 18 | 19 |
20 | 21 | 22 | 23 | 24 | 25 | 26 |
27 | 28 | 29 | 30 |
Tags
- 슬라이딩 윈도우
- Python
- 모던 JavaScript 튜토리얼
- 에라토스테네스의 체
- 누적 합
- 해시 테이블
- DFS
- 13164
- 정수론
- DP
- 브루트포스
- BFS
- JavaScript
- 투 포인터
- 정렬
- 그리디
- SSAFY
- 2357
- 수학
- 세그먼트 트리
- 싸피
- boj
- 구현
- 트리
- 문자열
- 애드 혹
- 그래프
- 이분 탐색
- 플로이드-워셜
- 맵
Archives
- Today
- Total
흙금이네 블로그
[BOJ] 14938 - 서강그라운드 (Python) 본문
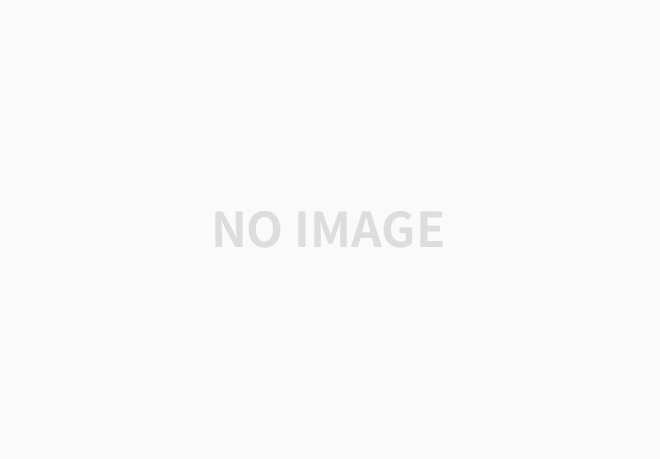
아이디어 #1
플로이드-워셜을 이용하여 지역 간 최소 거리를 갱신하고, 획득할 수 있는 최대 아이템 수를 계산한다.
풀이 #1
import sys
input = sys.stdin.readline
def solution():
n, m, r = map(int, input().split())
T = [0]+list(map(int, input().split()))
D = [[150000]*(n+1) for _ in range(n+1)]
for _ in range(r):
a, b, l = map(int, input().split())
D[a][b] = l
D[b][a] = l
for k in range(1, n+1):
D[k][k] = 0
for i in range(1, n):
for j in range(i+1, n+1):
if D[i][k]+D[k][j] < D[i][j]:
D[i][j] = D[j][i] = D[i][k]+D[k][j]
items = [0]*(n+1)
for i in range(1, n+1):
items[i] += T[i]
for j in range(i+1, n+1):
if D[i][j] <= m:
items[i] += T[j]
items[j] += T[i]
print(max(items))
solution()
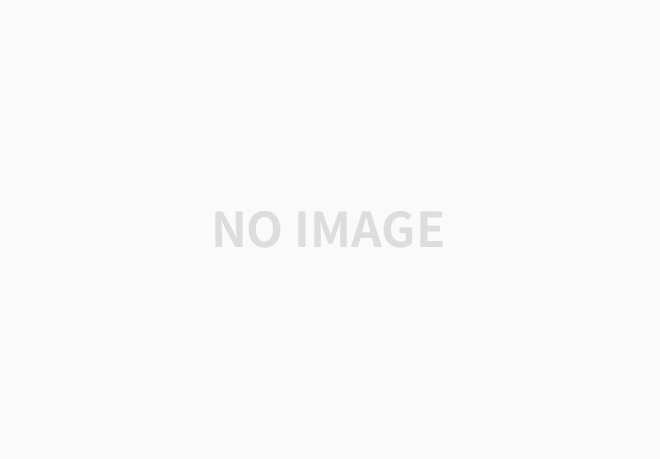
아이디어 #2
다익스트라를 이용하여 지역 간 최소 거리를 갱신하고, 획득할 수 있는 최대 아이템 수를 계산한다.
풀이 #2
import sys
from heapq import heappush, heappop
input = sys.stdin.readline
def solution():
n, m, r = map(int, input().split())
T = [0]+list(map(int, input().split()))
graph = [[] for _ in range(n+1)]
for _ in range(r):
a, b, l = map(int, input().split())
graph[a].append((l, b))
graph[b].append((l, a))
items = [0]*(n+1)
for i in range(1, n+1):
D = [150000]*(n+1)
heap = [(0, i)]
D[i] = 0
while heap:
d, idx = heappop(heap)
for next_d, next_idx in graph[idx]:
if d+next_d < D[next_idx]:
D[next_idx] = d+next_d
heappush(heap, (d+next_d, next_idx))
items[i] += T[i]
for j in range(i+1, n+1):
if D[j] <= m:
items[i] += T[j]
items[j] += T[i]
print(max(items))
solution()
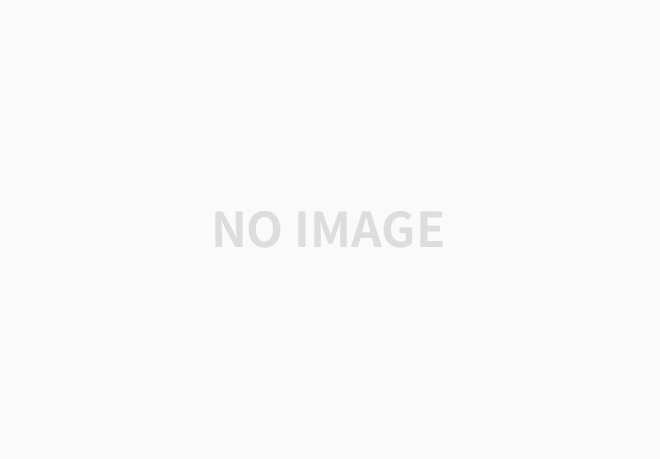
'알고리즘' 카테고리의 다른 글
[BOJ] 4991 - 로봇 청소기 (Python) (0) | 2023.04.05 |
---|---|
[BOJ] 2668 - 숫자고르기 (Python) (0) | 2023.04.03 |
[BOJ] 4159 - 알래스카 (Python) (0) | 2023.04.01 |
[BOJ] 21609 - 상어 중학교 (Python) (0) | 2023.04.01 |
[BOJ] 19238 - 스타트 택시 (Python) (0) | 2023.03.31 |